「Swiper」、Javascriptベースのプラグイン使ってみました。
React、Vueでも動かせて公式のサイトでもコードが載っており実装が簡単なのも魅力ですね。
インストールはこちら。
npm install swiper
npmからインストールしたいプロジェクトまでcdコマンドで移動し、コマンドを入力でOKです。
Swiperの使いたい機能をインポート
Swiperでは機能ごとにcssとモジュールを読み込みます。
今回はスライダーでよく使うナビゲーション(矢印)、ページネーション、オートプレイ、フェードインの切り替えを実装します。
完成系イメージは下記の2カラムで画像を表示します。
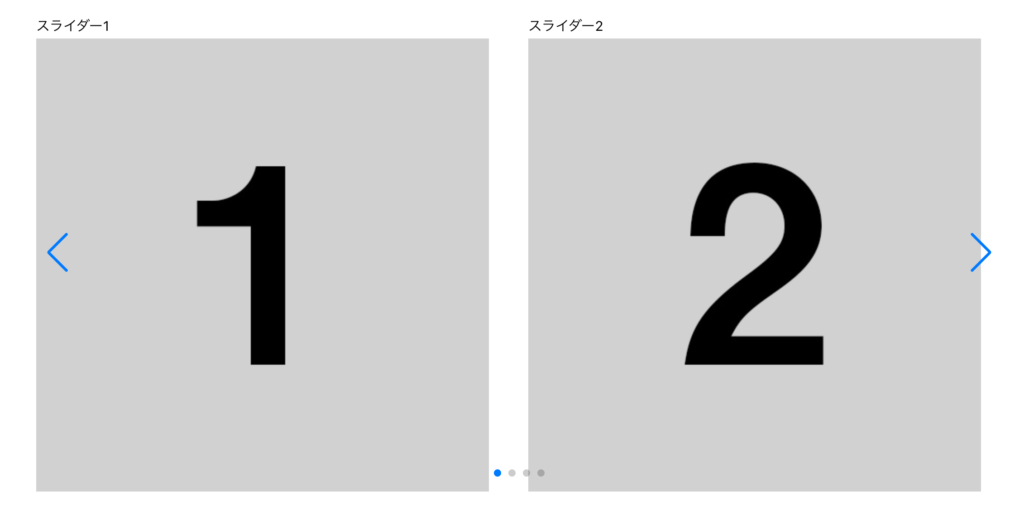
コンポーネントでのインポートの記述は下記です。
import { Swiper, SwiperSlide } from 'swiper/react';
//css
import "swiper/css";
import "swiper/css/navigation";
import "swiper/css/pagination";
import 'swiper/css/effect-fade';
//モジュール
import { Navigation, Pagination, EffectFade, Autoplay, } from 'swiper/modules';
それぞれの機能ごとにCSSとモジュールを読み込んでいます。これで機能を使うことができます。
注意:読み込むCSSについて
Google検索やChatGPTで導入方法を調べたのですが、CSSのインポートでこの記述を目にしました。
import 'swiper/swiper-bundle.min.css';
このインポート先は最新のものでは存在しないため読み込むことができず、エラーになります(2024年7月時点)。
以前にVueで開発した時はこの記述だったので、Swiperのバージョンが変わって読み込むパスも変わったのかもですね…?
Swiperのpachage.jsonを見てみましたがこちらにも記述はありませんでした。
(ちなみにswiperのnodeモジュール上のパスはこちら:node_modules/swiper/package.json)
注意なポイントですね。
実装
コンポーネントの全体は下記です。
import { Swiper, SwiperSlide } from 'swiper/react';
import "swiper/css";
import "swiper/css/navigation";
import "swiper/css/pagination";
import 'swiper/css/effect-fade';
import { Navigation, Pagination, EffectFade,Autoplay, } from 'swiper/modules';
import illust from '../../assets/images/sample_squre1.jpg';
import illust2 from '../../assets/images/sample_squre2.jpg';
export default function Illust() {
return (
<>
<h2 className='secTitle'>Illust</h2>
<Swiper
modules={[Navigation, Pagination, Autoplay, EffectFade]}
navigation={true}
pagination={{ clickable: true,}}
slidesPerView={1}
spaceBetween={20}
loop={true}
speed={1000}
autoplay={{
delay: 3000,
disableOnInteraction: false,
}}
breakpoints={{
751: {
slidesPerView: 2,
},
}}
>
<SwiperSlide>
<p>スライダー1</p>
<div><img src={illust} alt=""/></div>
</SwiperSlide>
<SwiperSlide>
<p>スライダー2</p>
<div><img src={illust2} alt=""/></div>
</SwiperSlide>
<SwiperSlide>
<p>スライダー3</p>
<div><img src={illust} alt=""/></div>
</SwiperSlide>
<SwiperSlide>
<p>スライダー4</p>
<div><img src={illust2} alt=""/></div>
</SwiperSlide>
</Swiper>
</>
);
}
「Swiper」というタグで全体を囲い、「SwiperSlide」が各スライドになります。
様々なオプションがSwiperタグについています。
オプションについては下記のサイトで細かく記載があります!探しやすいので参考にぜひ!
【最新】Swiperの使い方・カスタマイズを解説!サンプルやオプション15個付き ー基礎から応用までー
Swiper公式の方にもサンプルとReactやVueのコードつきで載ってるのでかなり便利です。
オプションを変数でまとめるには
上記のサンプルでは「Swiper」タグに直接オプションを記述しています。
他のhtmlと一緒になると全体のコードがみずらくなっていますね…。
Swiperではオプションを変数にまとめて渡すことができます。サンプルが下記です。
import { Swiper, SwiperSlide } from 'swiper/react';
import "swiper/css";
import "swiper/css/navigation";
import "swiper/css/pagination";
import 'swiper/css/effect-fade';
import { Navigation, Pagination, EffectFade,Autoplay, } from 'swiper/modules';
import illust from '../../assets/images/sample_squre1.jpg';
import illust2 from '../../assets/images/sample_squre2.jpg';
const swiperParams = {
modules: [Navigation, Pagination, Autoplay, EffectFade],
navigation: true,
pagination: { clickable: true,},
slidesPerView: 1,
spaceBetween: 20,
loop: true,
speed: 2000,
autoplay: {
delay: 5000,
disableOnInteraction: false,
},
breakpoints: {
751: {
slidesPerView: 2,
},
},
};
export default function Illust() {
return (
<>
<h2 className='secTitle'>Illust</h2>
<Swiper {...swiperParams}>
<SwiperSlide>
<p>スライダー1</p>
<div><img src={illust} alt=""/></div>
</SwiperSlide>
<SwiperSlide>
<p>スライダー2</p>
<div><img src={illust2} alt=""/></div>
</SwiperSlide>
<SwiperSlide>
<p>スライダー3</p>
<div><img src={illust} alt=""/></div>
</SwiperSlide>
<SwiperSlide>
<p>スライダー4</p>
<div><img src={illust2} alt=""/></div>
</SwiperSlide>
</Swiper>
</>
);
}
「swiperParams」という変数にSwiperのオプションを設定しています。
あとはこれを「swiper」タグにスプレット構文で{…swiperParams}と記述すればOKです!
かなり見やすくなりました!
フェードインでのスライダー切り替え
変数で記述したオプション部分です。1カラムで表示を切り替えるように変えています。
const swiperParams = {
modules: [Navigation, Pagination, Autoplay, EffectFade],
navigation: true,
pagination: {
clickable: true,
},
slidesPerView: 1,
spaceBetween: 20,
loop: true,
speed: 2000,
effect: 'fade',
fadeEffect: {
crossFade: true,
},
autoplay: {
delay: 5000,
disableOnInteraction: false,
},
breakpoints: {
751: {
slidesPerView: 1,
},
},
};
注意ポイントですが「effect: ‘fade’」 と「fadeEffect: { crossFade: true,}」というオプションをつけています。
「effect: ‘fade’」だけではスライドの切り替えがうまくいかないため、必ず「fadeEffect: { crossFade: true,}」をセットでつけましょう。
「slidesPerView:」を1にしていますが、2以上にしても画像が1つしか表示されません。何かやり方があるのかもしれませんが、表示がおかしくなってしまうので注意です。
まぁ…フェードの切り替えで画像2つ以上が変わるデザインは見たことない気もしますし見栄えもあまり良くないので、もしかしたら対応してないのかもですね…
まとめ
以上Swiperの基本的な使い方でした。
スライダーは使う機会が多いので他にいい使い方があれば書いていきたいと思います。